Sets a method to execute each frame,
before the Animation draws itself. The Animation class comes with
a number of step methods preset, or custom methods can be used.
Using predefined step methods
Using predefined step methods is easy (see example).
Included methods are:
- ELLIPSE
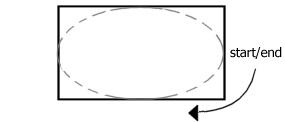
This method traces an ellipse clockwise, where the four sides
of the animation-rectangle define the four corners of the ellipse.
- SCALE
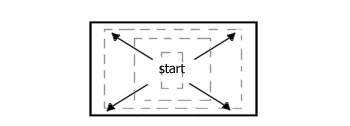
This method scales the image from its original
size to the size of the animation-rectangle, always keeping the
image in the center of the rectangle. (It doesn't scale nested
Animations.)
- BOX
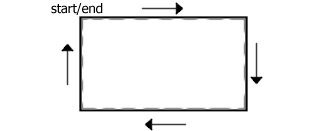
This method traces the animation box clockwise.
- BOUNCE
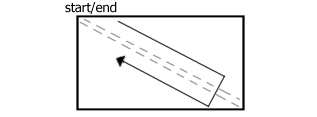
This method moves from the top-left to the
bottom-right, then back to the top-left.
- NOISE
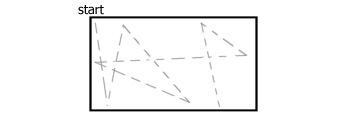
This method bounces around the animation-rectangle
in a pseudorandom fashion.
- NONE
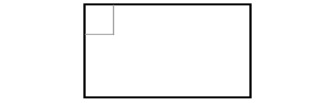
This method defines no motion: the animation stays in the top-left
corner..
Creating custom step methods
A step method is responsible for determining the location
of the Animation, given the percent complete that the Animation
currently is. Think of a custom step method as defining a motion
equation for the Animation to follow. (see example.)
Valid prototypes for a custom step method are
- int[] myMethod(int);
- int[] myMethod(float);
- float[] myMethod(int);
- float[] myMethod(float);
where the parameter and return value is:
Required parameter
A custom step method must receive the percent complete
that the Animation is at that step, either as a float
(25% complete is passed as 0.25) or as an int
(25% complete is passed as 25).
Required return value
A custom step method must return either int[]
or float[], where the 0th index is the
new x-position and the 1th index is the new y-position.
|